ARM Assembly for Embedded Applications, 5th ed.
|
Course Materials
- Table of Contents and Preface (5th ed.)
- PowerPoint Lecture Slide Decks (by chapter)
PowerPoint Lecture Slides
- Chapter 1: Introduction
- Chapter 2: Binary Number Systems
- Chapter 3: Writing Functions in Assembly
- Chapter 4: Copying Data
- Chapter 5: Integer Arithmetic
- Chapter 6: Making Decisions and Writing Loops
- Chapter 7: Manipulating Bits
- Chapter 8: Multiplication and Division Revisited
- Chapter 9: Getting Started with Floating Point
- Chapter 10: Floating-Point Emulation
- Chapter 11: Fixed-Point Reals
- Chapter 12: Multimedia Processing
- Chapter 13: Composite Data Types
- Chapter 14: Inline Code
- Chapter 15: Programming Peripheral Devices
- Programming Labs (by chapter)
Programming Labs (by chapter)
- ARM Instruction Worksheets
ARM Instruction Worksheets
- Textbook Errata Documents
Textbook Errata Documents
Documentation
Software
|
Pre-Recorded Lectures
|
32F429IDISCOVERY (Front)
(Part number STM32F429I-DISC1)
|
Note: This text and supporting materials were developed for a sophomore-level introductory course on assembly language programming, presented within the context of embedded applications.
Although most embedded applications are now written in a high-level language, they typically run on inexpensive processors that offer limited performance and functionality. For example,
many do not offer floating-point instructions or (in some cases) divide or multiply instructions. For that reason, embedded applications often require that time-critical portions be
implemented as functions written in assembly and called from a main program written in a language like C. This text follows that approach, presenting a number of techniques for writing
time-efficient code in assembly. The accompanying programming labs provide experience applying these techniques to the implementation of entertaining and informative applications, with
many that measure and report the number of processor clock cycles required to execute the assembly language functions or that demonstrate specific optimization techniques.
|
Page Revised: 03/07/24 17:13:51 PST
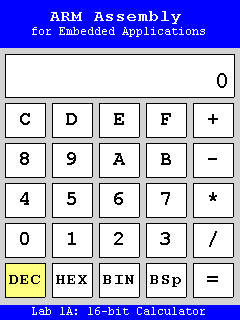
Chapter 1: Introduction to the program development environment
Code Revised: 02/14/21 19:35:44 PST
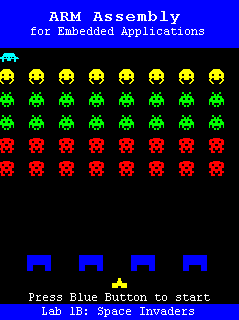
Chapter 1: Introduction to the program development environment
Code Revised: 12/07/21 10:40:03 PST
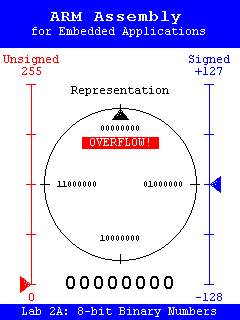
Chapters 1-2: Decimal to binary conversion, binary to decimal conversion, adding 1 in binary
Code Revised: 02/14/21 19:38:15 PST
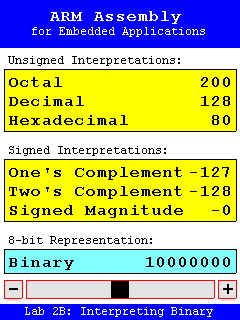
Chapters 1-2: Converting binary to octal, hex, decimal, 2's complement and signed magnitude
Code Revised: 01/03/22 09:21:53 PST
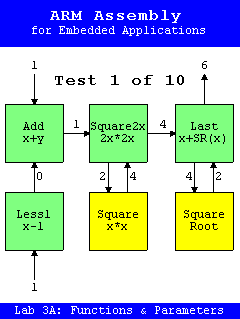
Chapters 1-3: Parameters, return value, & functions calling functions
Code Revised: 01/15/22 17:06:14 PST
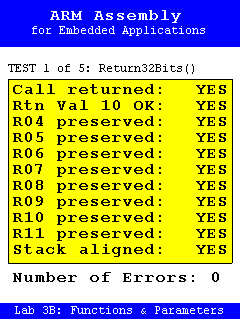
Chapters 1-3: Parameters, return value, & functions calling functions
Code Revised: 01/15/22 17:01:11 PST
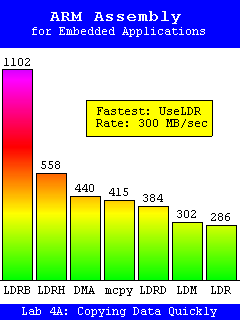
Chapters 1-4: Finding the fastest way to copy a large block of data
Code Revised: 08/20/22 16:58:30 PST
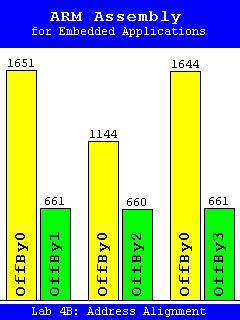
Chapters 1-4: Address alignment, execution time, and the .REPT and .ENDR directives
Code Revised: 02/14/21 18:37:26 PST
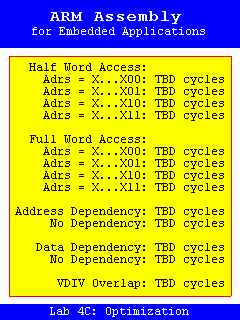
Chapters 1-4: Address alignment, dependencies, overlapped execution
Code Revised: 08/20/22 08:44:41 PST
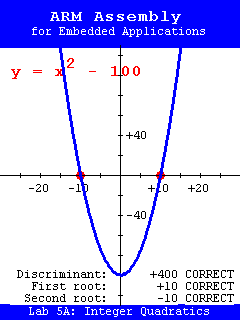
Chapters 1-5: Basic integer arithmetic, calling C function from assembly
Code Revised: 01/14/22 10:43:52 PST
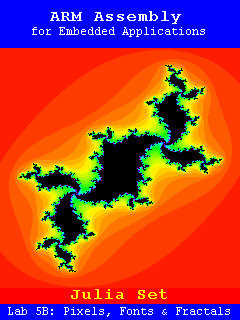
Chapters 1-5: Calculating the address of a pixel & indexing into a font table
Code Revised: 08/20/22 09:32:27 PST
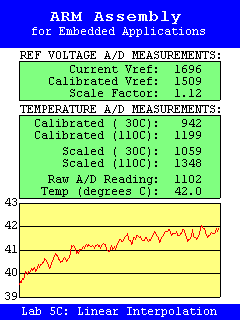
Chapters 1-5: Linear functions and interpolation using integer operands
Code Revised: 02/14/21 18:37:26 PST
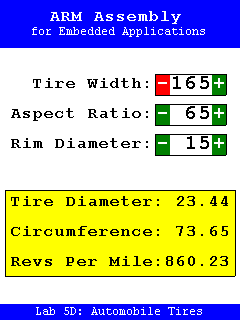
Chapters 1-5: Linear functions using integer operands
Code Revised: 02/14/21 19:47:07 PST
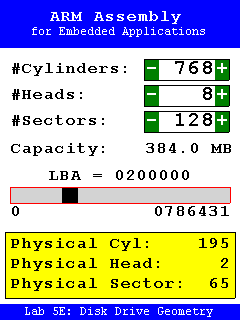
Chapters 1-5: Integer arithmetic
Code Revised: 04/27/21 13:28:38 PST
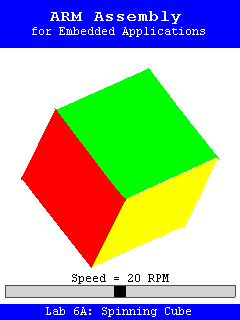
Chapters 1-6: 2D subscripting, nested loops,calling C functions from assembly
Code Revised: 02/14/21 18:37:26 PST
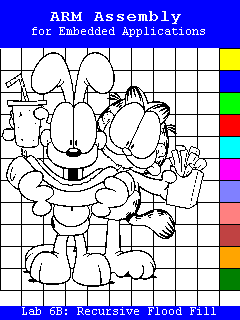
Chapters 1-6: Recursion, decisions, function calls, parameter passing
Code Revised: 06/18/21 20:13:17 PST
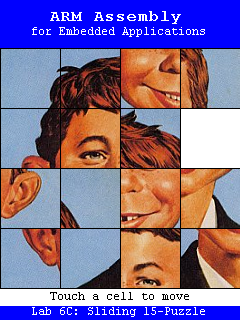
Chapters 1-6: Function calls, parameter passing, loops and pointers
Code Revised: 06/18/21 20:14:31 PST
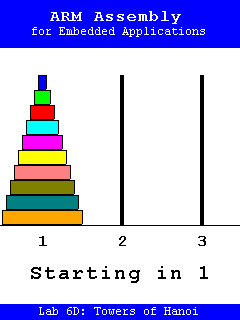
Chapters 1-6: Recursion, parameters, return value & nested functions
Code Revised: 08/20/22 09:33:30 PST
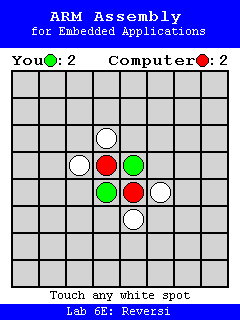
Chapters 1-6: Parameters, return value, compares, IT blocks & autoincrement
Code Revised: 08/20/22 09:33:51 PST
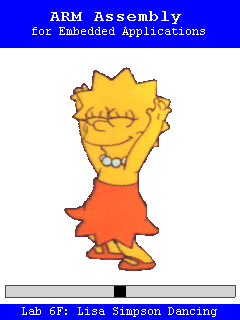
Chapters 1-6: One-dimensional subscripting, post-indexed addressing and loops
Code Revised: 06/18/21 20:04:46 PST
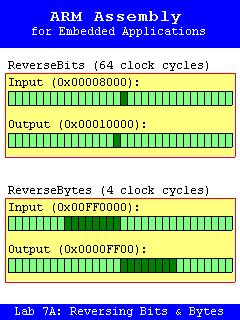
Chapters 1-7: Reversing the order of bits and bytes without using REV or RBIT instructions
Code Revised: 02/14/21 19:55:23 PST
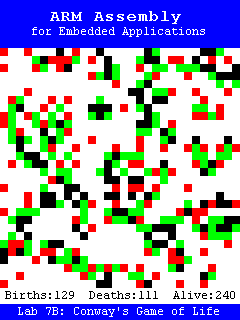
Chapters 1-7: Bit manipulation, shift and bitwise instructions, bit-banding
Code Revised: 08/20/22 09:34:23 PST
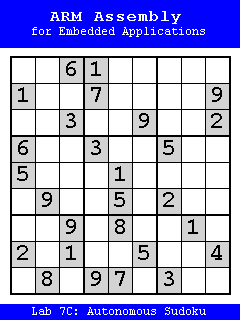
Chapters 1-7: Shift, bitwise and bitfield instructions; address calculation with pointers
Code Revised: 02/14/21 20:00:10 PST
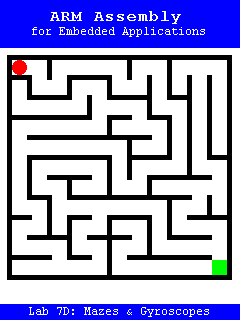
Chapters 1-7: Shift, bitwise and bitfield instructions; address calculation with pointers
Code Revised: 02/14/21 20:02:40 PST
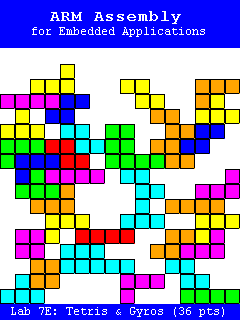
Chapters 1-7: Bit manipulation, shift and bitwise instructions, bit-banding
Code Revised: 08/20/22 09:34:50 PST
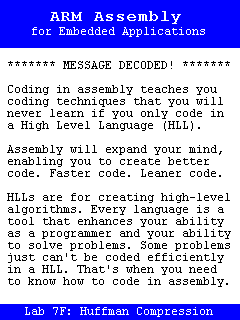
Chapters 1-7: Bitwise and shift instructions, bit-banding, loops
Code Revised: 02/14/21 20:05:54 PST
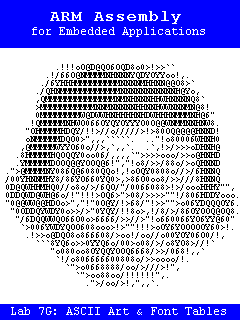
Chapters 1-7: Bit manipulation, shift and bitwise instructions, nested loops
Code Revised: 06/18/21 20:17:28 PST
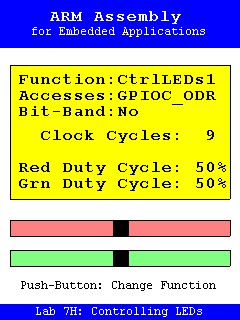
Chapters 1-7: Bit manipulation, shift instructions, bitfields, bit-banding
Code Revised: 02/14/21 20:07:50 PST
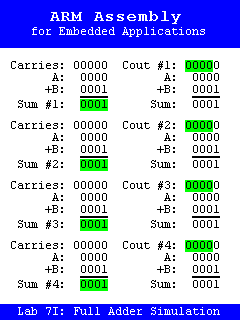
Chapters 1-7: Integer arithmetic, bitwise and shift instructions
Code Revised: 08/20/22 09:35:28 PST
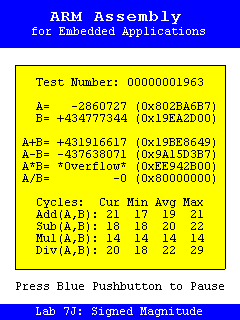
Chapters 1-7: Bitwise and bitfield operations, arithmetic with signed magnitude integers
Code Revised: 06/21/21 09:44:51 PST
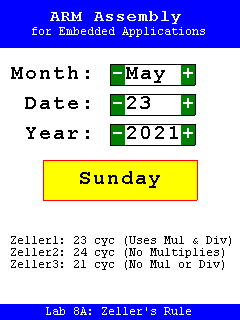
Chapters 1-8: Using instruction sequences to replace division and multiplication by a constant
Code Revised: 05/23/21 10:57:31 PST
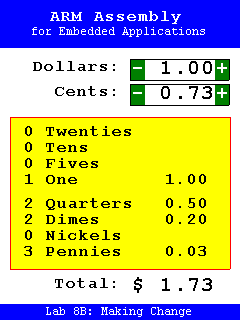
Chapters 1-8: Using instruction sequences to replace division and multiplication by a constant; accessing structures members from assembly
Code Revised: 02/14/21 18:37:27 PST
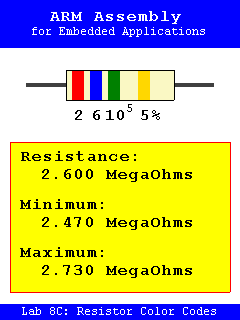
Chapters 1-8: Using instruction sequences to replace division and multiplication by a constant
Code Revised: 02/25/21 11:53:20 PST
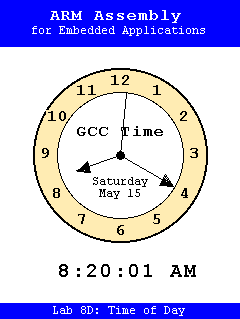
Chapters 1-8: Using instruction sequences to replace division and multiplication by a constant
Code Revised: 05/16/21 09:13:51 PST
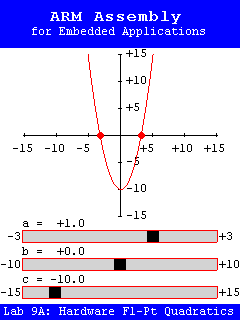
Chapters 1-9: Solving quadratic equations with hardware floating-point
Code Revised: 08/20/22 09:36:12 PST
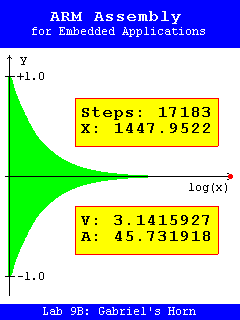
Chapters 1-9: Floating-point computation, numerical integration
Code Revised: 08/20/22 09:36:28 PST
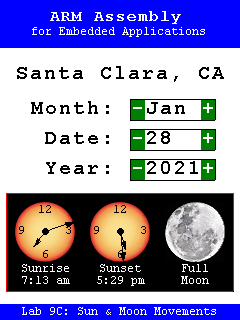
Chapters 1-9: Floating-point arithmetic, pointer to structure
Code Revised: 08/20/22 09:36:50 PST
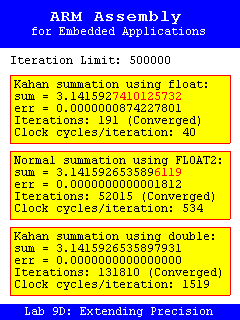
Chapters 1-9: Floating-point arithmetic, series approximations, Kahan summation, double floats
Code Revised: 02/14/21 20:15:58 PST
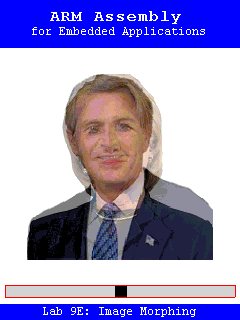
Chapters 1-9: Floating-point arithmetic and conversions, bit manipulation, loops
Code Revised: 06/18/21 20:09:11 PST
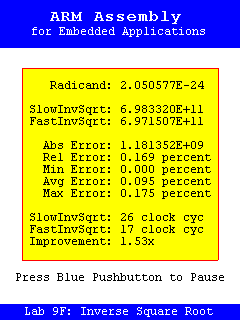
Chapters 1-9: Floating-point arithmetic
Code Revised: 06/21/21 08:20:57 PST
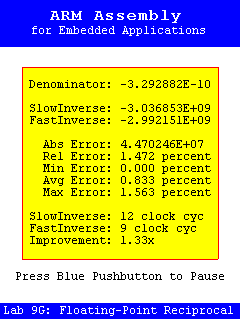
Chapters 1-9: Floating-point arithmetic
Code Revised: 06/21/21 08:20:34 PST
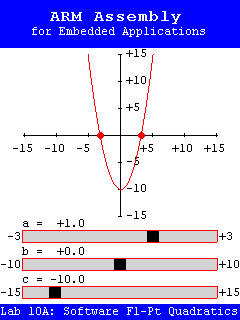
Chapters 1-10: Solving quadratic equations with software floating-point
Code Revised: 08/20/22 09:37:27 PST
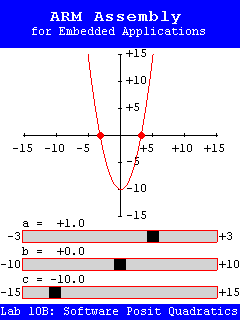
Chapters 1-10: Solving quadratic equations with software posit reals
Code Revised: 08/20/22 09:37:45 PST
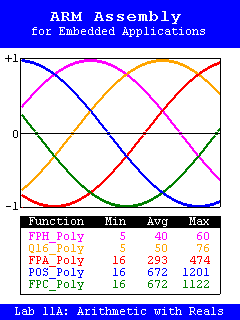
Chapters 1-11: Comparing performance of H/W and S/W real arithmetic to evaluate Taylor series approximations
Code Revised: 06/09/21 15:56:01 PST
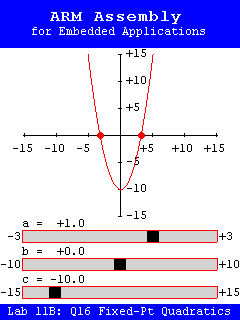
Chapters 1-11: Solving quadratic equations with fixed-point reals
Code Revised: 08/20/22 09:38:09 PST
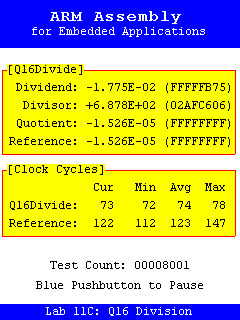
Chapters 1-11: Implementing division for Q16 fixed-point reals
Code Revised: 06/09/21 15:39:01 PST
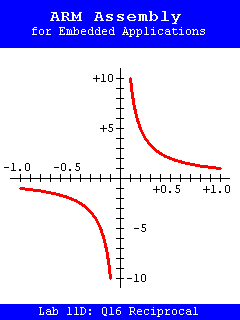
Chapters 1-11: Q16 arithmetic, reciprocal division, Newton's method
Code Revised: 03/09/21 15:46:55 PST
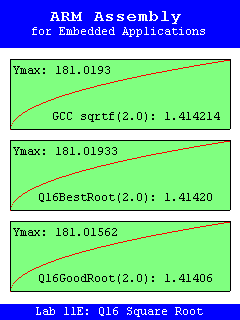
Chapters 1-11: Q16 and integer square roots
Code Revised: 06/04/21 15:12:44 PST
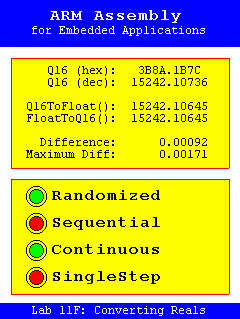
Chapters 1-11: Q16 and floating-point representations, bit manipulation
Code Revised: 02/14/21 18:37:26 PST
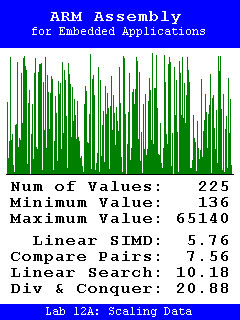
Chapters 1-12: SIMD processing, finding min and max values of an array.
Code Revised: 02/14/21 18:37:26 PST
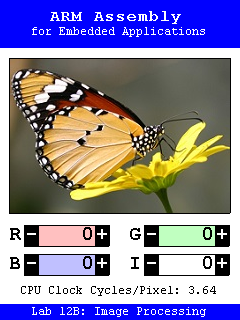
Chapters 1-12: SIMD processing, Saturating Arithmetic, Composite Data Types and Inline Assembly
Code Revised: 02/14/21 20:21:13 PST
Workspace for GNU Toolchain, including run-time library and include files required for all lab assignments
Code Revised: 12/08/22 19:26:24 PST